The spread operator allows an iterable, such as an array or string, to be expanded in places where zero or more arguments (for function calls) or elements and the syntax for this is three periods (…)
In this article, I will explain the use case of the spread operator in JavaScript, so let’s jump right to it.
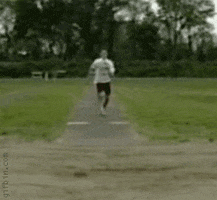
Use Cases
Adding the elements of an existing array into a new array
let newarr = ["Red", "Yellow"];
let otherarr = ["Blue", ...newarr, "Green"]
console.log(otherarr); // ["Blue", "Red", "Yellow", "Green"];
So let’s explain what is happening here, newarr is an array containing two strings Red and Yellow, then the second array otherarr contains two strings and the newarr, usually, when we add this array without the three periods operator it will add the new array into the other array. but as we have the spread operator it will add the element of the array into the other array.
You can pass elements of an array as arguments to a function
function addThreeNumbers(x,y,z){
return x+y+z;
}
let args = [1,2,3];
addThreeNumbers(...args); // 6
Another use case is that you can pass an element of an array as a function. in the above example, we have a function addThreeNumbers with three parameters x,y,z which return the sum of these three numbers. Now we have an array with three values 1,2,3. In a normal state, we can pass three arguments to the function but with the help of the spread operator, we just pass the array with the three-dot operator (…), which will spread out the array to each element of the function.
Note: If we have a fourth element in the array it going to ignore the fourth element in the array, like the example below.
function addThreeNumbers(x,y,z){
return x+y+z;
}
let args = [1,2,3,4];
addThreeNumbers(...args); // 6
Copy Arrays
let arr1 = [1,2,3];
let arr2 = [...arr1]
So if we have arr1 we can use the spread operator to copy the array into the second array.
Concatenate array
let arr1 = [1,2,3];
let arr2 = [5,6,7];
arr1.concat(arr2); // [1,2,3,5,6,7]
arr1 = [...arr1 ...arr2]; // [1,2,3,5,6,7]
Another use case is that we can concatenate arrays together, normally how we do this, is we use the concat method to concatenate arrays together but with the spread operator, we can concatenate arrays too.
Reference
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Spread_syntax
Leave a Reply